Cracking the Docker CLI: How to Grasp Container Management Commands
When you are new to Docker (or Podman, or nerdctl, or alike), the number of commands to study might be truly overwhelming.
Docker tries to control the complexity of its CLI by employing a neat grouping technique. The first thing you see after running docker help
is a list of so-called Management Commands - umbrella entry points gathering the actual commands by their area of responsibility. But even this list is no short, and it's actually a list of lists!
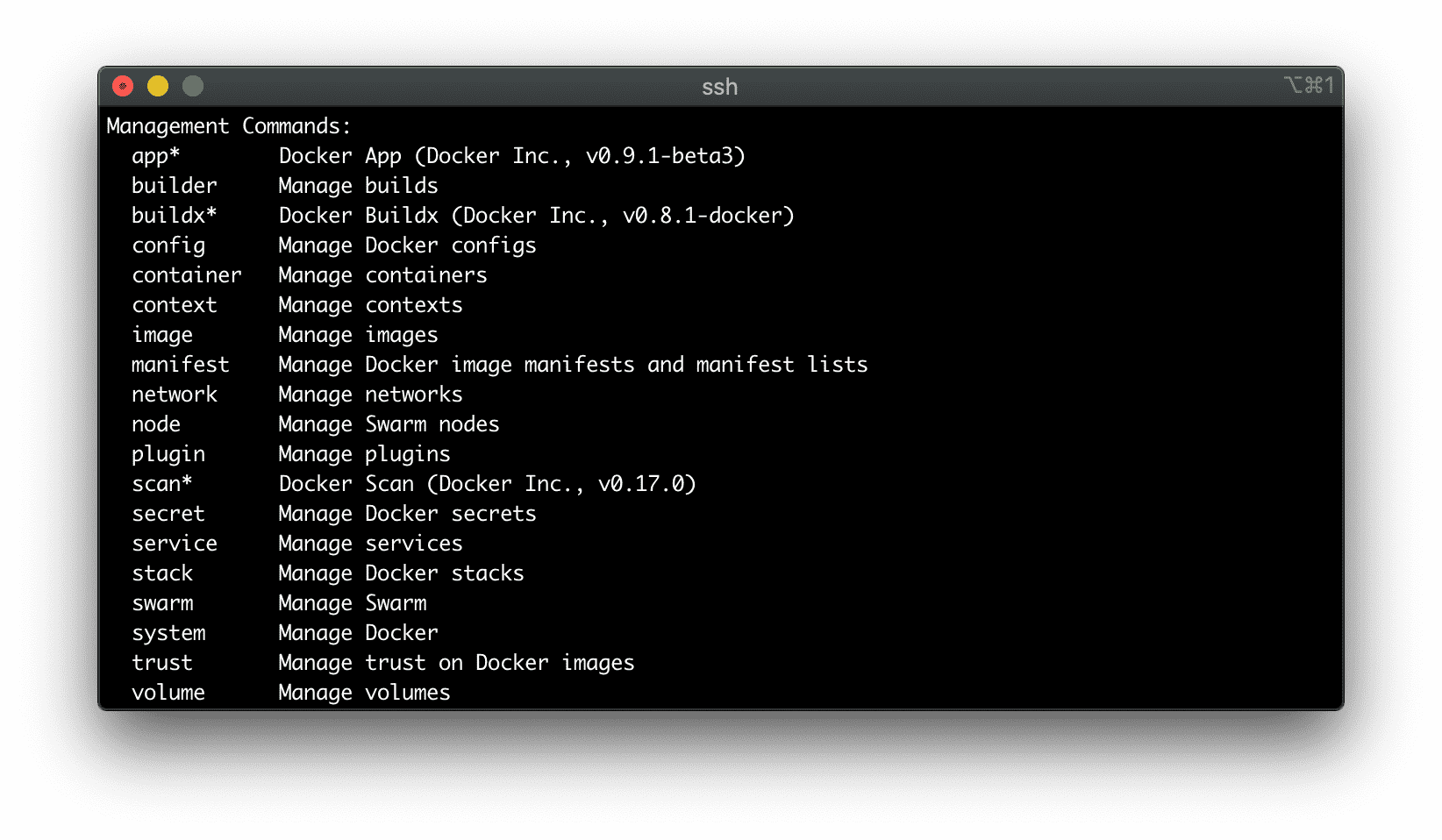
Also, historically, many commands are known through their shorter but vaguer aliases - for instance, you'd rather stumble upon docker ps
than docker container list
in the wild. So, the struggle is real 🤪
However, there might be a way to internalize (at least some of) the most important Docker commands without the brute-force memorization!
The goal of this article is to show how a tiny bit of understanding of the containers' nature can help you master Docker's CLI, starting from the most foundational group of commands - commands to manage containers.